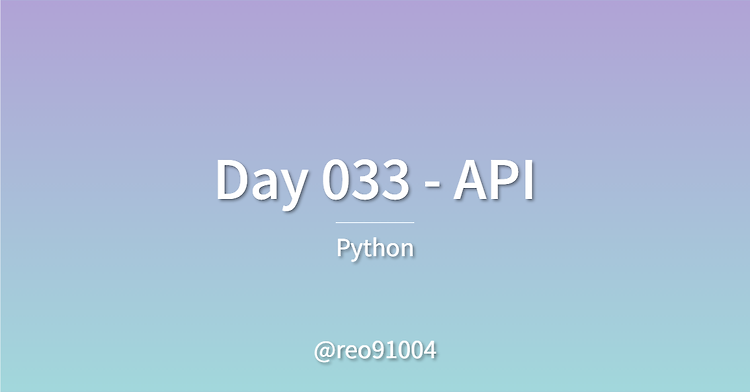
시작하며
API는 Application Programming Interface로, 이를 이용해 소프트웨어를 생성하거나 외부 시스템과 상호작용 할 수 있습니다. 이용자들은 누구나 API의 규칙에 맞게 외부 시스템에 데이터를 요청해 응답받아 데이터를 사용할 수 있습니다.
대충 레스토랑에 우리가 주방에 직접 들어가서 음식을 만들 수는 없지만, 메뉴에 있는 음식을 주문할 수 있다고 생각하면 됩니다.
API에서 가장 중요한 것은
API 엔드포인트
입니다. 쉽게 말해서 주소를 알아야 한다는 것인데, 보통 github.io같은 URL로 표현됩니다.
API Request
도 중요합니다. 데이터를 요청해 빼 오는 것인데, 정식 절차를 밟아야 데이터를 건네주는데 여기서 정식 절차가 API라고 할 수 있습니다.
가장 간단한 예시는 국제 정거장의 현재 위치 API입니다. 해당 사이트에 들어가면 http://api.open-notify.org/iss-now.json
링크가 존재하는데, 이 링크가 바로 API이며 접속하면 현재 국제 정거장의 위치가 나옵니다.
접속 시 나오는 좌표
JSON Viewer Pro 확장 프로그램을 설치하면 조금 더 예쁘게 볼 수 있습니다.
한번 파이썬에서 실행시켜 봅시다.
import requests
response = requests.get(url="http://api.open-notify.org/iss-now.json")
print(response)
>>> <Response [200]>
JSON 형식으로 나오지 않고 200이 출력되는 이유는 정상적으로 처리되었다는 응답 코드의 일종입니다. 잘못된 링크를 넣으면 출력되는 404 NOT FOUND처럼요!
미니 프로젝트
이번에도 프로젝트라고 하기도 뭣한 초초미니 프로젝트를 한번 해보겠습니다. 위에서 말한 ISS API와, 일출/일몰을 알려주는 API를 활용해
ISS가 제 위치에 접근하고, 지금이 어둡다면
이메일을 발송하는 프로그램을 만들어 보겠습니다.
코드 전문입니다.
import requests
from datetime import datetime
import smtplib
# 1. 나의 위치 설정
# 본인의 위도와 경도는 https://www.latlong.net/Show-Latitude-Longitude.html 여기서 알 수 있습니다!
MY_LAT = 37.645341
MY_LONG = 126.628729
# 일출/일몰 시간을 알기 위한 파라미터 설정
parameters = {
"lat": MY_LAT,
"lng": MY_LONG,
"formatted": 0, # 24시간 형식
}
# 메일 Class
class SendMail:
def __init__(self) -> None:
self.my_email = "your_email"
self.password = "your_password"
self.send_mail()
def send_mail(self):
with smtplib.SMTP("smtp.gmail.com") as connection:
connection = smtplib.SMTP("smtp.gmail.com")
connection.starttls()
connection.login(user=self.my_email, password=self.password)
connection.sendmail(
from_addr=self.my_email,
to_addrs="your_email",
msg=f"Subject:Hey!\n\nLook over your head!"
)
# 2. 내 지역의 일몰 시간 설정!
def is_night():
response_sun = requests.get("https://api.sunrise-sunset.org/json", params=parameters)
response_sun.raise_for_status()
data = response_sun.json()
sunrise = int(data['results']['sunrise'].split("T")[1].split(":")[0])
sunset = int(data['results']['sunset'].split("T")[1].split(":")[0])
time_now = datetime.now().hour
if time_now > sunset or time_now < sunrise:
return True
# 3. 현재 ISS의 위치 가져오기!
def iss_overhead():
response_iss = requests.get(url="http://api.open-notify.org/iss-now.json")
response_iss.raise_for_status() # 에러 응답 코드 출력
data = response_iss.json()
longitude = data['iss_position']['longitude']
latitude = data['iss_position']['latitude']
iss_position = (longitude, latitude)
# 만약 내 머리 위를 봤을때 ISS를 볼 수 있는 각도라면
if MY_LAT-5 <= iss_position <= MY_LAT+5 and MY_LONG-5 <= iss_position <= MY_LONG+5:
return True
# 일몰이 지났고, 일출 전인 밤이라면
if iss_overhead() and is_night():
send_mail = SendMail()
어려웠던 점?
sunrise = int(data['results']['sunrise'].split("T")[1].split(":")[0])
처럼 split
함수를 좀 여러 번 겹처쓰는게 익숙하지 않았습니다.
부록
참고문헌
Sunset and sunrise times API - Sunrise-Sunset.org
Reverse Geocoding Tool Convert Lat Long to Address
'◎ Python > Udemy Python' 카테고리의 다른 글
Day 038 - 구글 시트에 운동 기록 (1) | 2024.03.08 |
---|---|
Day 037 - 습관 추적기 프로젝트 (0) | 2024.03.08 |
Day 036 - 주식시장 알림 프로젝트 (1) | 2024.03.08 |
Day 032 - SMTP와 Datetime 활용 (2) (0) | 2024.03.08 |
Day 032 - SMTP와 Datetime 활용 (0) | 2024.03.08 |
자기계발 블로그